PHP Mastery: Unlocking the Power of Web Development | by Emperor Brains | May, 2024
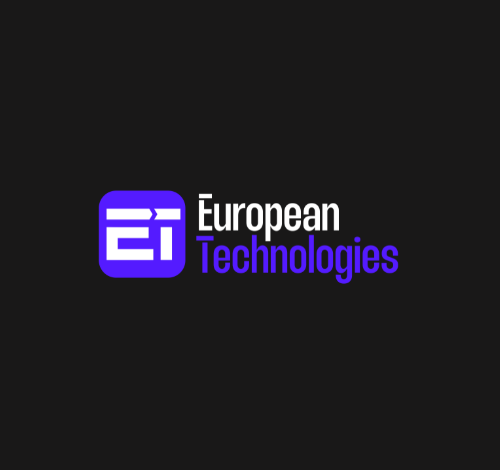
PHP is an open-source language created by Rasmus Lerdorf in 1994. It has evolved significantly since its inception and is now a powerful tool for building complex web applications. PHP is known for its ease of use, flexibility, and compatibility with various databases and servers.
Simplicity and Ease of Use
- PHP syntax is easy to understand, making it an ideal choice for beginners.
- It integrates seamlessly with HTML, allowing developers to embed PHP code within HTML tags.
Cross-Platform Compatibility
- PHP runs on various platforms, including Windows, Linux, and macOS.
- It is compatible with most web servers like Apache and Nginx.
Database Integration
- PHP supports numerous databases, including MySQL, PostgreSQL, Oracle, and more.
- It provides built-in functions to interact with databases, making data retrieval and manipulation straightforward.
Open Source
- PHP is free to use, which makes it accessible to a wide range of developers and businesses.
- A large and active community contributes to continuous improvements and a vast library of extensions.
Scalability and Performance
- PHP is highly scalable and can handle large applications and websites.
- It is designed to execute scripts on the server, resulting in fast load times for users.
Versatility
- PHP can be used for various types of web development, from small websites to large-scale web applications.
- It supports a wide range of frameworks and content management systems (CMS), including Laravel, Symfony, WordPress, and Joomla.
Cost-Effective
- Being open-source, PHP reduces the cost of development.
- It has a vast ecosystem of free resources, tutorials, and tools.
Security
- PHP has built-in features to protect against common web vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
- Regular updates and a vigilant community help maintain its security standards.
Rich Ecosystem
- PHP has an extensive repository of libraries and frameworks that streamline development processes.
- The availability of pre-built modules and plugins accelerates development time.
Understand the Basics
- Learn the fundamental syntax and structure of PHP.
- Practice embedding PHP code within HTML.
Database Interaction
- Gain proficiency in connecting to databases and executing queries.
- Learn to use PDO (PHP Data Objects) for secure and efficient database interaction.
Object-Oriented Programming (OOP)
- Master OOP concepts to write modular and reusable code.
- Understand classes, objects, inheritance, and polymorphism.
Error Handling and Debugging
- Implement robust error handling to manage exceptions and errors gracefully.
- Use debugging tools and techniques to troubleshoot and optimize code.
Security Best Practices
- Sanitize and validate user inputs to prevent security vulnerabilities.
- Use prepared statements to protect against SQL injection attacks.
Performance Optimization
- Optimize code for better performance by minimizing server load and reducing execution time.
- Use caching techniques to enhance page load speed.
Setting Up the Environment
- Install a local server environment like XAMPP or WAMP.
- Set up a code editor or integrated development environment (IDE) such as VS Code or PHPStorm.
Creating a Basic PHP Script
- Start with a simple script to understand PHP syntax and basic operations.
<?php
echo "Hello, World!";
?>
Connecting to a Database
- Establish a connection to a MySQL database using PDO.
<?php
$dsn = 'mysql:host=localhost;dbname=testdb';
$username = 'root';
$password = '';try {
$pdo = new PDO($dsn, $username, $password);
echo "Connected to the database successfully!";
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
Building a CRUD Application
- Create a basic CRUD (Create, Read, Update, Delete) application to manage data.
- Develop forms to handle user input and display data from the database.
Implementing MVC Architecture
- Use the Model-View-Controller (MVC) architecture to separate business logic, presentation, and data.
- Implement a simple MVC framework or use an existing one like Laravel or Symfony.
Deploying the Application
- Test the application thoroughly on a local server.
- Deploy the application to a live server using FTP or a version control system like Git.
Mastering PHP opens up a world of opportunities in web development. Its simplicity, versatility, and robust features make it an excellent choice for both beginners and experienced developers. By following best practices and continuously learning, you can harness the full potential of PHP to build dynamic and interactive web applications.
At Emperor Brains, we specialize in leveraging cutting-edge technologies like PHP to deliver top-notch web development solutions. Our team of skilled developers is dedicated to creating innovative and efficient web applications that meet the unique needs of our clients. Whether you’re looking to build a new website from scratch or enhance an existing one, Emperor Brains is your trusted partner in web development.
Visit us at Emperor Brains to learn more about our services and how we can help you unlock the power of PHP for your next project.