Open a popup on a link – JavaScript – SitePoint Forums
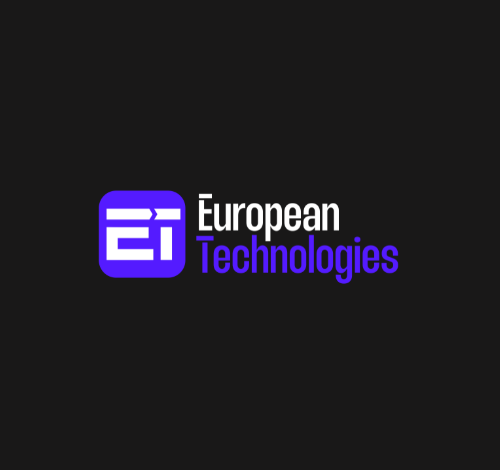
Good morning,
I tried to make a popup on the link in my footer:
<li><a class="show-popup" href="#popup1">Payment Method</a></li>
and I get this result, how can I solve this problem?
https://codepen.io/aaashpnt-the-sans/pen/eYaEGPO
I added this in my html
<div id="popup1" class="popup-container">
<div class="popup-box">
<h1 style="font-size: 1.9em;">Fonctionnalités</h1>
<p>- Connectez-vous au système à l’aide de la session.</p>
<p>- Ajouter/Modifier et mettre à jour/Supprimer des catégories.</p>
<p>- Ajouter/Modifier et mettre à jour/Supprimer des produits.</p>
<p>- Ajouter/Modifier et mettre à jour/Supprimer des clients.</p>
<p>- Les données client sont utilisées lors de la création des commandes pour lesquelles cette
commande est créée.</p>
<p>- Ajouter/Modifier et mettre à jour/Supprimer des administrateurs afin que plusieurs
administrateurs puissent gérer ce
système de point de vente.
</p>
<p>- Créer des commandes.</p>
<p>- Passer les commandes.</p>
<p>- Récapitulatif de la commande.</p>
<p>- Dashboard Analytics.</p>
<p>* Vous recevrez un guide complet lors de votre achat.</p>
<button class="close-btn">OK</button>
</div>
</div>
and this in my css
/* Popup */
body {
min-height: 100vh;
}
.popup-container {
position: fixed;
left: 0;
top: 0;
width: 100%;
height: 100vh;
background: rgba(0, 0, 0, 0.3);
display: flex;
justify-content: center;
align-items: center;
opacity: 0;
pointer-events: none;
overflow: auto;
z-index: 99;
}
.popup-container.active {
opacity: 1;
pointer-events: auto;
transition: 0.4s ease;
}
.popup-container .popup-box {
width: 500px;
background: #f2f2f2;
border-radius: 6px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
padding: 30px;
transform: scale(0);
}
.popup-container.active .popup-box {
transform: scale(1);
transition: 0.4s ease;
transition-delay: 0.25s;
}
.popup-box h1 {
color: #333;
line-height: 1;
}
.popup-box p {
color: #333;
margin: 12px 0 20px;
font-size: 1.4em;
}
.popup-box .close-btn {
width: 100%;
height: 45px;
background: #ef6e12;
border-radius: 6px;
border: none;
outline: none;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
cursor: pointer;
font-size: 18px;
color: #f2f2f2;
font-weight: 500;
}
And js
const showPopup = document.querySelectorAll(".show-popup");
const closeBtn = document.querySelectorAll(".close-btn");
showPopup.forEach(function (popup) {
popup.addEventListener("click", function () {
var modalTarget = this.getAttribute("href");
document.querySelector(modalTarget).classList.add("active");
});
});
closeBtn.forEach(function (close) {
close.addEventListener("click", function () {
this.closest(".popup-container").classList.remove("active");
});
});
here’s some js errors showing in that codepen which I guess is because al assets are not available. It seems to be this one stopping the popup from working though.
Uncaught SyntaxError: Identifier 'products' has already been declared
If I comment this line out:
//import {products} from "./items.js";
The popover modal now shows but you need a higher z-index.
.popup-container {
z-index: 100;
}
Still needs a bit of work though.
2 Likes
Thank you, it works now. The css is not easy for me I tinker a lot and there are details that I cannot resolve like the shopping basket in the navbar the numbering I cannot manage to indicate above the shopping basket.
I also now have a shift in my footer and I cannot align the 3 payment method images.
I don’t know which tag we should use to lower an element.
I tried with this and the images come down well but they are no longer aligned
.flex {
display: flex;
flex-wrap: wrap;
}
The html for those brands is invalid as you you can’t have a div as a direct child of a ul
. All content in a ul
must be inside li
tags.
It should be like this:
<div class="footer-col">
<h4>Online Store</h4>
<ul>
<li><a href="#onlinestore">Order Now</a></li>
<li><a class="show-popup" href="#popup1">Payment Method</a></li>
<li class="footer-brands">
<i class="fa-brands fa-cc-visa fa-2xl" style="color: #FFD43B;"></i>
<i class="fa-brands fa-cc-mastercard fa-2xl" style="color: #FFD43B;"></i>
<i class="fa-brands fa-cc-stripe fa-2xl" style="color: #FFD43B;"></i>
</li>
</ul>
</div>
Avoid using classes like .flex as that makes no sense especially if you later change to grid or some other position.
I called it this:
<li class="footer-brands">
Then the css becomes:
/* footer aligner moyen de paiement */
.footer-brands {
display: flex;
flex-wrap: wrap;
gap:1rem;
margin:2rem 0;
}
On another matter note that on your landing page you have this rule.
.landing-page {
height: 100vh;
width: 100%;
etc...
}
That is cutting your content off completely on smaller height windows (such as in codepen or mobiles or small desktops).
You need to use min-height instead of height so that content can grow in it really needs to.
1 Like
Try like this:
.rightItem{
display:flex;
flex-direction:column;
align-items:center;
}
.totalItem{
margin-bottom:-9px;
}
The html stays the same.
1 Like
It’s great, thank you, it works.
It works now I have the numbering above I think it’s better.
My css is becoming very long and difficult to navigate.
Consistency is the key and you need to organise things into the sections that they refer to which is why you have to be careful with your class names as they need to identify the sections without tying them to a style or behaviour.
There are lot of good articles about but heres one form the MDN network which should always be a first stop for checking css things.
Hi @cdevl3749,
Sorry this isn’t much of a contribution, but I did spot something in your code earlier.
Template strings can be used over multiple lines so this,
itemContainer.innerHTML +=
` <div class="card">`+
` <article class="cardImg">`+
` <img src="https://www.sitepoint.com/community/t/open-a-popup-on-a-link/./img/${productImg}" alt="">`+
`</article> `+
` <div class="itemDescContainer">`+
`<article class="itemDesc">`+
`<h1 class="itemName">${productName}</h1>`+
`<p class="itemPrice">${productPrice}$</p>`+
` </article> ` +
`<div class="addtocart" id="addtocart${id}")'>`+
`<i class="fa-solid fa-cart-shopping cart"></i>`+
` </div>`+
` </div>`+
`</div>`
Can be refactored to this
itemContainer.innerHTML +=
`
<div class="card">
<article class="cardImg">
<img src="https://www.sitepoint.com/community/t/open-a-popup-on-a-link/./img/${productImg}" alt="">
</article>
<div class="itemDescContainer">
<article class="itemDesc">
<h1 class="itemName">${productName}</h1>
<p class="itemPrice">${productPrice}$</p>
</article>
<div class="addtocart" id="addtocart${id}")>
<i class="fa-solid fa-cart-shopping cart"></i>
</div>
</div>
</div>
`
Only a small thing, but should help a bit with readability.
2 Likes
Many thanks to you, it works
1 Like