Web Page View Counter with Local Storage. | by Monish Nule | Apr, 2024
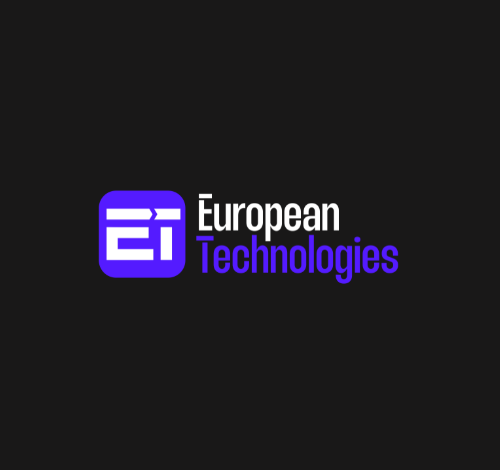
In the Article I explain Step by step Guide to implement your view Counter which will increment for every refresh. There are many ways we can do it. This Local Storage stores to local Storage of the User. This means that, the User will know how many time he/she had viewd the page. rather than collecting total views globally, you can achieve this too with Upstash/redis. Here is the link for Andrew’s Step by step guide to it ( you need to learn Nextjs for that).
So let’s dive into the JSX View Counter with Local Storage.
Any project with JavaScript or with library can apply this.
- Create a PageViewCounter.jsx file. (We are using JSX to import/export this component anywhere we want.
- useEffects, useStates.
import React, { useEffect, useState } from "react";
3. Create a JSX Component : using UseStates and usEffects.
const PageViewCounter = () => {
const [viewCount, setViewCount] = useState(0);return (
);
};
export default PageViewCounter;
4. Define increamentView(), and call it.
const PageViewCounter = () => {
const [viewCount, setViewCount] = useState(0);useEffect(() => {
// Function to increment the view count
const incrementView = () => {
// Check if 'viewCount' key exists in local storage
if (localStorage.getItem('viewCount')) {
// If it exists, increment the count
let count = parseInt(localStorage.getItem('viewCount')) + 1;
localStorage.setItem('viewCount', count);
setViewCount(count);
} else {
// If it doesn't exist, set the count to 1
localStorage.setItem('viewCount', 1);
setViewCount(1);
}
};
// Call the incrementView function to increment the count when the component mounts
incrementView();
}, []);
return (
);
};
export default PageViewCounter;
5. Now Return the ViewCount as you desire in formate you like, use Tailwind css to Style it too.
const PageViewCounter = () => {
const [viewCount, setViewCount] = useState(0);useEffect(() => {
// Function to increment the view count
const incrementView = () => {
// Check if 'viewCount' key exists in local storage
if (localStorage.getItem('viewCount')) {
// If it exists, increment the count
let count = parseInt(localStorage.getItem('viewCount')) + 1;
localStorage.setItem('viewCount', count);
setViewCount(count);
} else {
// If it doesn't exist, set the count to 1
localStorage.setItem('viewCount', 1);
setViewCount(1);
}
};
// Call the incrementView function to increment the count when the component mounts
incrementView();
}, []);
return (
<div>
<p className="text-xs"><span>{viewCount}</span> Views </p>
<p className="text-xs">bragging your Storage for Views</p>
</div>
);
};
export default PageViewCounter;
This is Simple way, you can understand how we apply the Logic in JS.
Now You can Use it any Project. But remember it is storing it Locally.
here the Final Code : Full Code to Refer
Here is my personal Website built on Next JS : https://monishnule.dev/